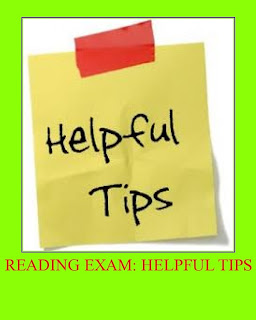
Pointer
· Pointer is a variable that represents the location of a data item.
· Address operator (&) : It evaluates the address of its operand.
· Indirection Operator(*): Used to access data item referenced by a pointer.
Example:
Main()
{
Int a=3;
Int *ptr;
Ptr=&a;
Printf(“%d %u” , *ptr, ptr);
}
Pointer to an array
· A pointer can be used to reference an array.
· The name of an array will work as the pointer to its first element.
Main()
{
Int a[]= { 1, 2 , 3, 4};
Int *ptr =a;
For(int i=0; i<4;i++)
{
Printf(“%d”,* ptr+i);
}
Arrays of Pointers
· A group of pointers are stored in an array.
· Arrays of pointers can be used to store multidimensional arrays
Datatype *array[size];
Structures:
· A collection of heterogeneous elements.
· In structure memory will be allocated for each element.
Struct student {
Char name[20];
Int mark;
};
Union
· Similar to structures, A collection of heterogeneous elements.
· Memory will be allocated for largest member only. Other members have to share this memory.
union student {
Char name[20];
Int mark;
};